Since our launch of the Mojo programming language on May 2nd, more than 120K+ developers have signed up to use the Mojo Playground and 19K+ developers actively discuss Mojo on Discord and GitHub. Today, we’re excited to announce the next big step in Mojo’s evolution: Mojo is now available for local download – beginning with Linux systems, and adding Mac and Windows in coming releases.
While the Mojo Playground provides an easy hosted introduction to the language, the local Mojo toolchain empowers developers to accomplish much more. Local developer tools provide access to the full power of Mojo, including a full set of compiler features and IDE tools that make it easy to build and iterate on Mojo applications.
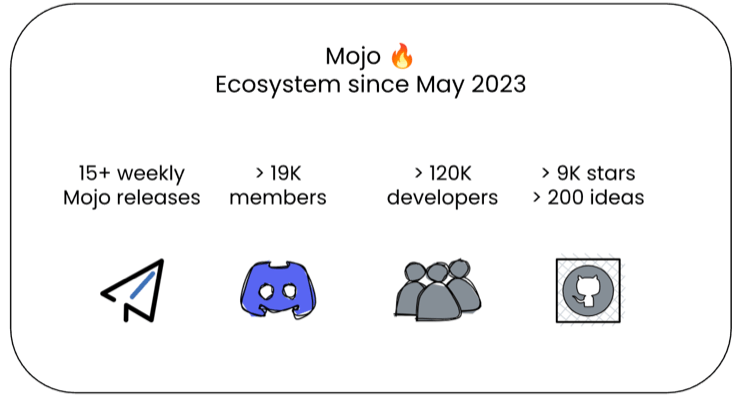
In this blog post, we’ll take a high-level tour of what’s inside the Mojo SDK. First, let’s quickly review what Mojo is and how it can benefit you.
Mojo: a high performance 'Python++' language for compute
Mojo is a new programming language for AI developers that will grow into being a superset of Python over time. It already supports integrating with arbitrary Python code seamlessly and has a scalable programming model to target performance-critical systems, including accelerators (e.g. GPUs) that are pervasive in AI.
Mojo meets you where you are today, and enables you to progressively adopt new features to enable high performance where you need it. Mojo combines the best of dynamic and static languages together, and can achieve up to 68,000x the performance of Python today. You can read more about Mojo’s origin story in our docs, but let’s recap a few important benefits that Mojo unlocks for users:
- Write everything in one language: Mojo meets AI developers where they are, combining the usability of Python with the systems programming features that cause developers to have to reach for C, C++, or CUDA. Your research and deployment teams can now work in a common codebase, streamlining the research to production workflow.
- Unlock Python performance: Python is pervasive, but not the right tool for tasks that require high performance or exotic hardware. Mojo enables high performance on CPUs, as well as support for exotic accelerators like GPUs and ASICs, providing performance on par with C++ and CUDA.
- Access the entire Python ecosystem: Mojo provides full interoperability with the Python ecosystem, making it seamless to use the Python libraries while taking advantage of Mojo’s features and performance benefits. For example, you can seamlessly intermix NumPy and Matplotlib with your Mojo code.
- Upgrade your AI workloads: Mojo integrates tightly with the Modular AI Engine, empowering you to easily extend your AI workloads with custom operations, including pre-processing, post-processing operations and high-performance mathematical algorithms. You can integrate kernel fusion, graph rewrites, shape functions, and more.
As we’ve shown in past blog posts, with simple code changes to existing Python code, you can get significant (>68000x) speedups with Mojo for compute-bound workloads. If you are a Python programmer, check out this blog post for an easy introduction to performance optimizations with Mojo.
Mojo is now available for download to your computer, but Mojo is a lot more than a compiler.
The Mojo Toolbox
The first release of the Mojo SDK comes with everything you need to easily develop Mojo programs. This includes the following tools:
- mojo driver: provides a shell (for read-eval-print-loop or REPL), and allows you to build and run Mojo programs, package Mojo modules (including support for the 🔥 extension!), generate docs, and format code
- Extension for Visual Studio Code (VS Code): supports various productivity features such as syntax highlighting, code completion, and more
- Jupyter kernel: supports building and running Mojo notebooks, including Python code
- Debugging support (coming soon): step into and inspect running Mojo programs, even intermixing C++ and Mojo stack frames
This initial release of the SDK supports X86/Linux systems, and we will expand to additional operating systems, hardware, and tool features in upcoming updates.
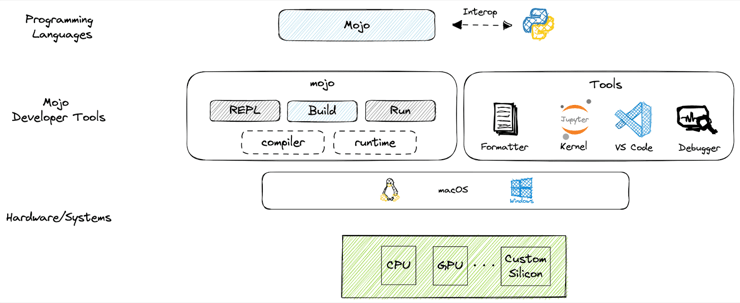
Now, let’s take a look at each of the tools available in the current release of the SDK.
Mojo Driver
Just as with Python, you can start programming in a REPL by running the mojo command. Here is an example that calculates Euclidean distance in Mojo from our previous blog post:
What’s more, Mojo allows you to build statically-compiled executables that you can deploy without any dependencies. For example, you can compile and run the hello.🔥 program from our examples repository as follows:
A 22 kB statically-compiled binary is pretty cool, and is made possible by Mojo’s tight dependency management.
Visual Studio Code Extension
Visual Studio Code is one of the most popular IDEs in the world, and Mojo supports it directly with an official extension on the Visual Studio Marketplace. This provides Mojo support for a number of developer productivity features:
- Syntax Highlighting
- Diagnostics and Fixits
- Definitions and References
- Hover help
- Formatting
- Code Completion
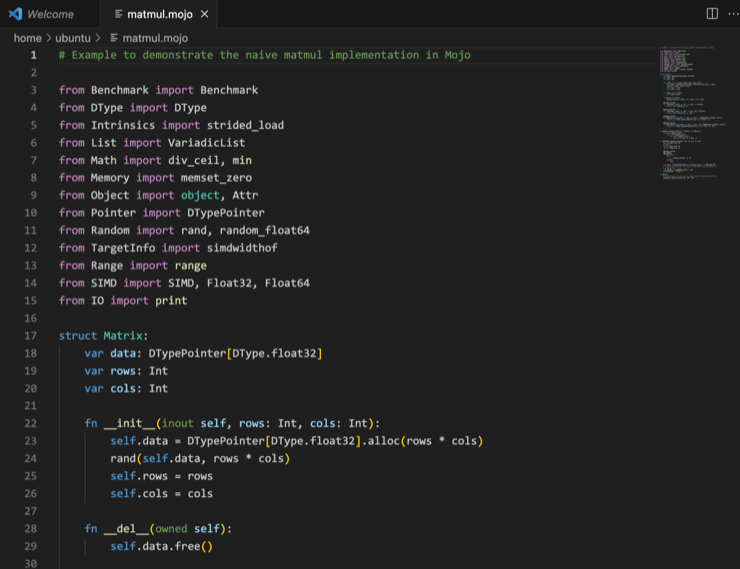

The language server and extension verifies Mojo code and displays errors and warnings inline:

The extension also supports hover help, showing embedded doc strings for API quick reference:

We will share more about the feature set in a future blog post. If you have any feedback or you like the extension, please leave a review!
Jupyter Integration
Jupyter provides a powerful environment for interactive development. Mojo includes a Jupyter kernel so you can get started right away with Jupyter notebooks. We’ve also shared all the notebooks from the Mojo Playground here on GitHub (see the README for details).
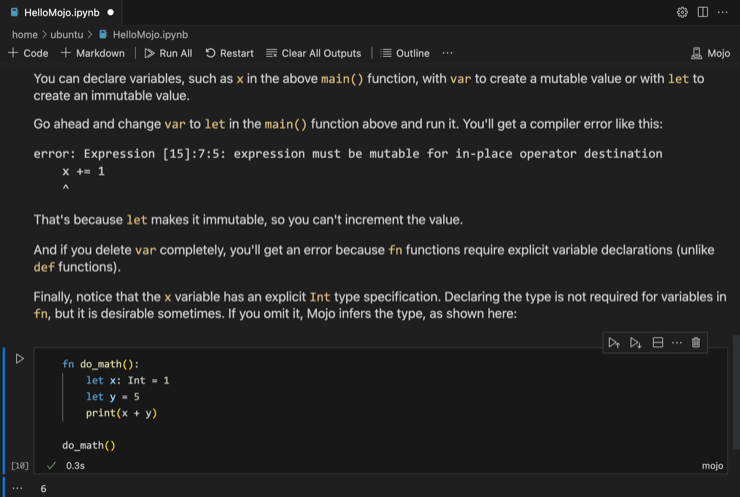
Debugging Support (coming soon)
In an upcoming release, we’ll add an interactive debugging experience that works in Visual Studio Code and via the LLDB command line interface. Not only that, Mojo’s debugger is able to seamlessly debug hybrid Mojo/C/C++ code in the same debug session, empowering developers even further when working with highly specialized code.
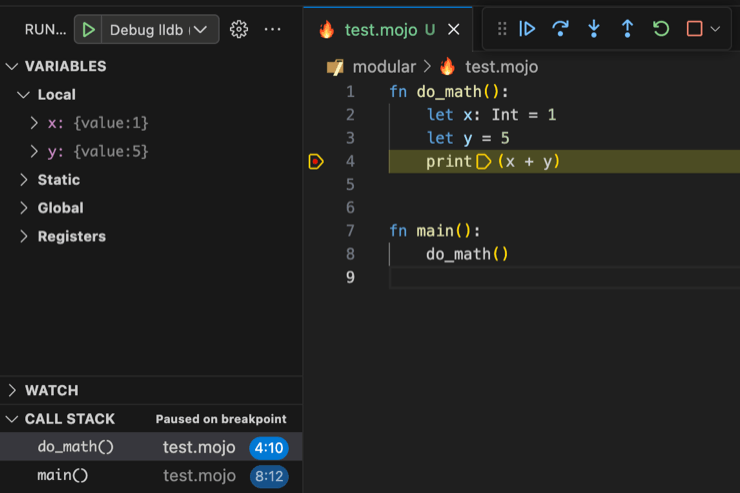
The Amazing Mojo Community ❤️🔥
We are thrilled about the creative and passionate developers that are getting in on the ground floor of Mojo development to build exciting things and we hope that the Mojo SDK makes this easier, collaborative and more fun. Some examples we’ve seen include:
- Blogger Maxim Zaks has implemented multiple tree data structures in Mojo and published a blog post and some preliminary benchmarks
- GitHub user MadAlex1997 built an N-Dimensional Array Implementation in Mojo
If you’re building something in Mojo, please join one of our communities (below) and share what you're working on. It's a great time to blog about your experiences and share projects.
More to come! 🚀
The first release of Mojo is now available for local development. We are eager for you to try it out and share your feedback. But this is just the beginning – we are continuing to drive language improvements, and will start open sourcing parts of Mojo later this year.
To learn more about Mojo and get involved:
- Get started with downloading Mojo
- Head over to the docs to read the programming manual and learn about APIs
- Explore the examples on GitHub
- Join our Discord community
- Contribute to discussions on the Mojo GitHub
- Report feedback, including issues on our GitHub tracker
Until then 🔥!
The author would like to extend a special thank you to all the engineers at Modular who worked on the Mojo SDK.